Overview
EventsPlus+ is a desktop address book application catered for busy university students to manage their contacts, events, project meetings and ad-hoc events efficiently. EventsPlus+ aims to simplify some of the more time-consuming administrative tasks such as coordinating location and date of ad-hoc events using a Command Line Interface (CLI). The user interacts with it using a CLI, and it has a GUI created with JavaFX. It is written in Java, and has about 10 kLoC.
Summary of contributions
-
Code contributed: [RepoSense]
-
Major enhancement: added location-related functionality
-
What it does: The two features added provide the following: 1. the ability to visually display the faculty location of a contact on a map and 2. the ability to generate a (random) meeting location for events or meetings and display this location on a map.
-
Justification: Events cannot take place without a location set in place. Adding location-related functionality with respect to a contact’s faculty and events improves the user experience significantly by providing the user one-stop location functionality without having to exit the application at all.
-
Highlights: A Faculty field is added to the Person class which is crucial given that this application is primarily targeted toward university students and thus such a field is necessary. Selecting which particular Google Maps API to be integrated into the application required careful consideration (the Embed API was eventually chosen for its flexibility with the JavaFX web browser). In the long run, the implementation of this foundational functionality allows for further development of other location-related features such as generating directions or generating convenient meeting locations (based on faculty locations) which is one of the features to be done in V2.0.
-
Credits: Google Maps Embed API was used for the map display.
-
-
Other contributions:
-
Minor enhancements:
-
Added a separate Location Display tab to display location-related information instead of using pre-existing Webpage tab: (Pull request #134)
-
Application opens maximized at launch: (Pull request #176)
-
Added simple default landing pages (when application is first launched) for Location Display tab and Webpage tab: (Pull request 134)
-
Wrote and modified tests to increase coverage by 4.4%: (Pull request 191)
-
-
Project management:
-
Documentation:
-
Community:
-
Tools:
-
Integrated Google Maps API into the project: (Pull request: #134)
-
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Show Faculty Location : showLocation
Shows the location of the person’s (identified by index number) faculty in the the location display panel.
Format: showLocation INDEX
Examples:
-
showLocation 5
Suggested Meeting Location : generateLocation
Creates a randomly suggested location in NUS to meet up for an event (uniquely identified by date and event’s index number).
Format: generateLocation d/EVENT_DATE i/EVENT_INDEX
Examples:
-
generateLocation d/2018-04-01 i/1
Generate timing for a group’s event/meeting : generateTiming
Generates a possible meeting timing for a group’s event/meeting based on all member’s schedule and availability.
Format: generateTiming group/GROUP_NAME
Examples:
-
generateTiming group/CS2103Group
|
Generate convenient location for an event/meeting : generateConvenientLocation
This command builds on the existing generateLocation command, however, instead of generating a random location it will generate a location based on the people attending the event (i.e. members in the group or people tagged to the event).
Thus this can be used within the context of a group’s events or the user’s own personal events.
Format: generateConvenientLocation group/GROUP_NAME d/EVENT_DATE i/EVENT_INDEX
(for group events) or
generateConvenientLocation d/EVENT_DATE i/EVENT_INDEX
(for user’s personal events)
Examples:
-
generateConvenientLocation group/CS2103Group d/2018-09-30 i/1
-
generateConvenientLocation d/2018-09-30 i/1
|
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Show Person’s faculty location visually
Current Implementation
The displaying of the a Person
's faculty is facilitated by the newly added Faculty
field in the Person
class. This
Faculty
field is defined as a separate class (akin to other classes constituting the Person
class such as Email
and Address
).
During the adding of Person
's to EventsPlus+'s address book, the Faculty
class will ensure that the argument passed in is that of
a valid NUS faculty. It does this via an inner enum class called Faculties
which stores as constants the only accepted Faculty
argument values. The isInEnum
method is used to check that the argument passed already exists as one of the constants.
The valid arguments allowed are namely:
-
SOC (School of Computing)
-
FOS (Faculty of Science)
-
YLLSOM (Yong Loo Lin School of Medicine)
-
FOD (Faculty of Dentistry)
-
BIZ (NUS Business School)
-
SDE (School of Design and Environment)
-
FOE (Faculty of Engineering)
-
FOL (Faculty of Law)
-
YSTCOM (Yong Siew Toh Conservatory of Music)
-
FASS (Faculty of Arts and Social Sciences)
A "-" response is also allowed as it is possible that EventsPlus+'s address book may indeed have contacts who are not students of NUS at the current point in time.
The command used to display the faculty visually is showLocation
.
In addition to the Faculty
field, the showLocation command is assisted by Google Maps Embed API that allows for locations
to be displayed visually in a separate tab, Location Display Tab. Google Maps uses unique Place IDs to identify locations
on the map. For each faculty, these place IDs are stored in a newly created class EmbedGoogleMaps
in the logic component of the
application. Together with the Google Maps API key, the place ID allows for the generation of the exact spot of the person’s
faculty to be displayed in the Location Display Tab.
When the (correct) showLocation
command is input together with the index of the individual whose faculty location is to be displayed,
the index is first checked to determine if it is correct (more than 0 and not larger than the size of the list of contacts
in EventsPlus+'s addressbook). Following this, the appropriate person is obtained from the FilteredPersonList
(this is so as to allow
the displaying of the contact’s faculty location even if the list is filtered).
A event, FacultyLocationDisplayChangedEvent
, also newly created for this showLocation command, is posted and is then handled by TabPanel
(which switches to the Location
Display Tab) and subsequently by LocationDisplayPanel
. The status message will show "Selected person: [INDEX]. Faculty location successfully displayed."
If the contact has no faculty, a default location - University Hall - is displayed on the map.
The above-mentioned information can be represented in the following sequence diagram:
Generate a random meeting location for event
The generation of a random meeting location primarily builds upon the visual display of a Person
's faculty. However
there are a few key differences in the process that one needs to take note of.
The command used to generate this possible meeting location is generateLocation
.
In order to generate a random location for an event, the user needs to input (in addition to the command) the event date and event index. This is to detect the unique event (because some events may have the same name) and to ensure that this event is currently in EventsPlus+.
Command parsing is handled in a similar manner as other commands but there exists a specific GenerateLocationCommandParser
to specifically parse
these kind of commands. The GenerateLocationCommand object created will then consist of two fields:
-
Event date
-
Event index
The values for these fields are based on the input given by the user. These values are checked for validity as per other event-related
commands before the object is created. Following this, EventsPlus+ is checked to determine whether this event does
exist. If it does exist, the event name is retrieved and stored in the GenerateCommandLocation
object.
The status message indicates that a meeting location (it will show the meeting name too) has been generated, if not it indicates to the user
that such as event does not exist.
A random meeting location is generated based on 7 locations (more will be added in future iterations) that have their Google Maps Place IDs stored in the EmbedGoogleMaps
class.
A call to the method that generates this location (more specifically, returns the Place ID of the location) is made in the execute
method of GenerateLocationCommand.
Following this, a event, RandomMeetingLocationGeneratedEvent
, newly created for this generateLocation command, is posted and is then handled by TabPanel
(which switches to the Location
Display Tab) and subsequently by LocationDisplayPanel
.
For simplicity, only the logic and event handling sections of the sequence diagram are shown below for the generateLocation
command:
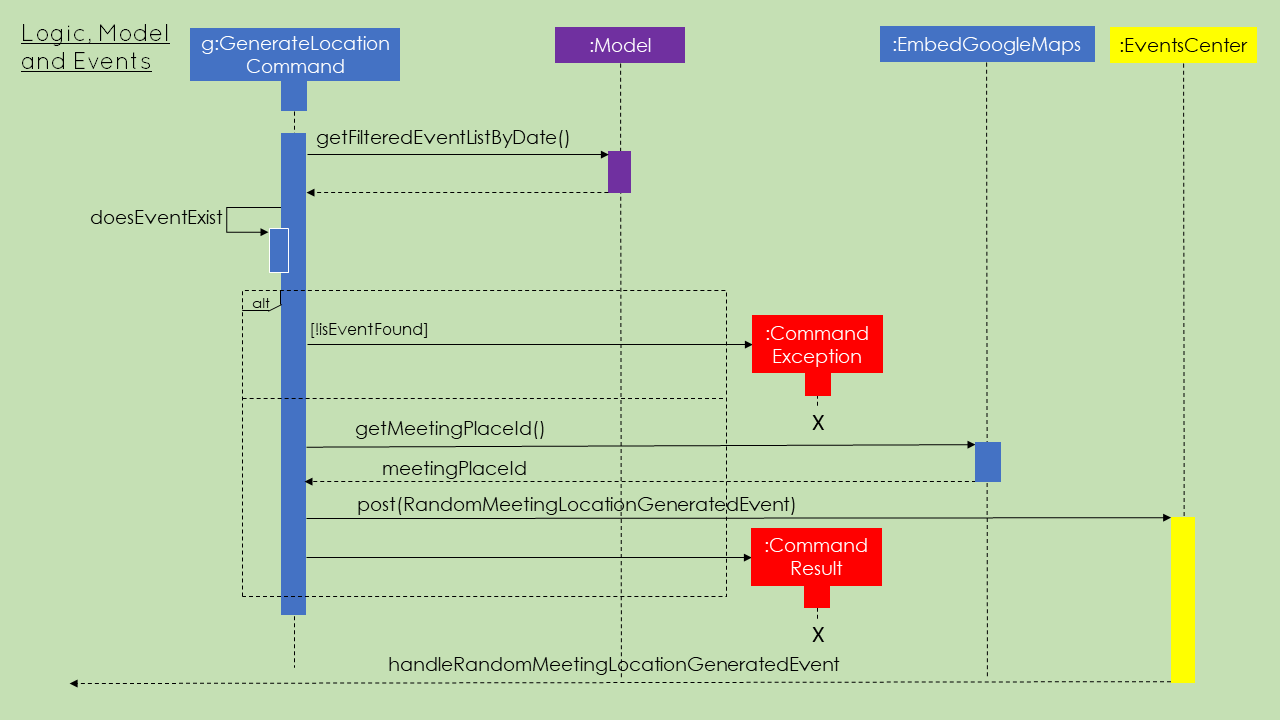